Understanding Streams and I/O in C++
In C++, streams are fundamental concepts that facilitate data flow between sources, destinations, and the computer. A stream is essentially an infinite sequence of characters that moves from a source to a destination, making it a powerful abstraction for handling input and output (I/O) operations in programming.
Streams in C++
- A Stream: In C++, a stream is defined as an infinite sequence of characters that flow from a source to a destination. Streams can either be input streams, which bring data into the program from an external source, or output streams, which send data from the program to an external destination.
- Input Stream: An input stream brings data from a source, such as a keyboard or a file, into the computer. The data is processed by the program as it flows into it.
- Output Stream: An output stream carries data from the computer to a destination, such as a screen or a file, allowing the program to display or store its results.
Common Stream Objects: cin
and cout
cin
: Thecin
object stands for “common input” and is an input stream object typically associated with the standard input device, which is usually the keyboard. It allows the program to receive user input.cout
: Thecout
object stands for “common output” and is an output stream object typically associated with the standard output device, which is usually the screen. It allows the program to display information to the user.
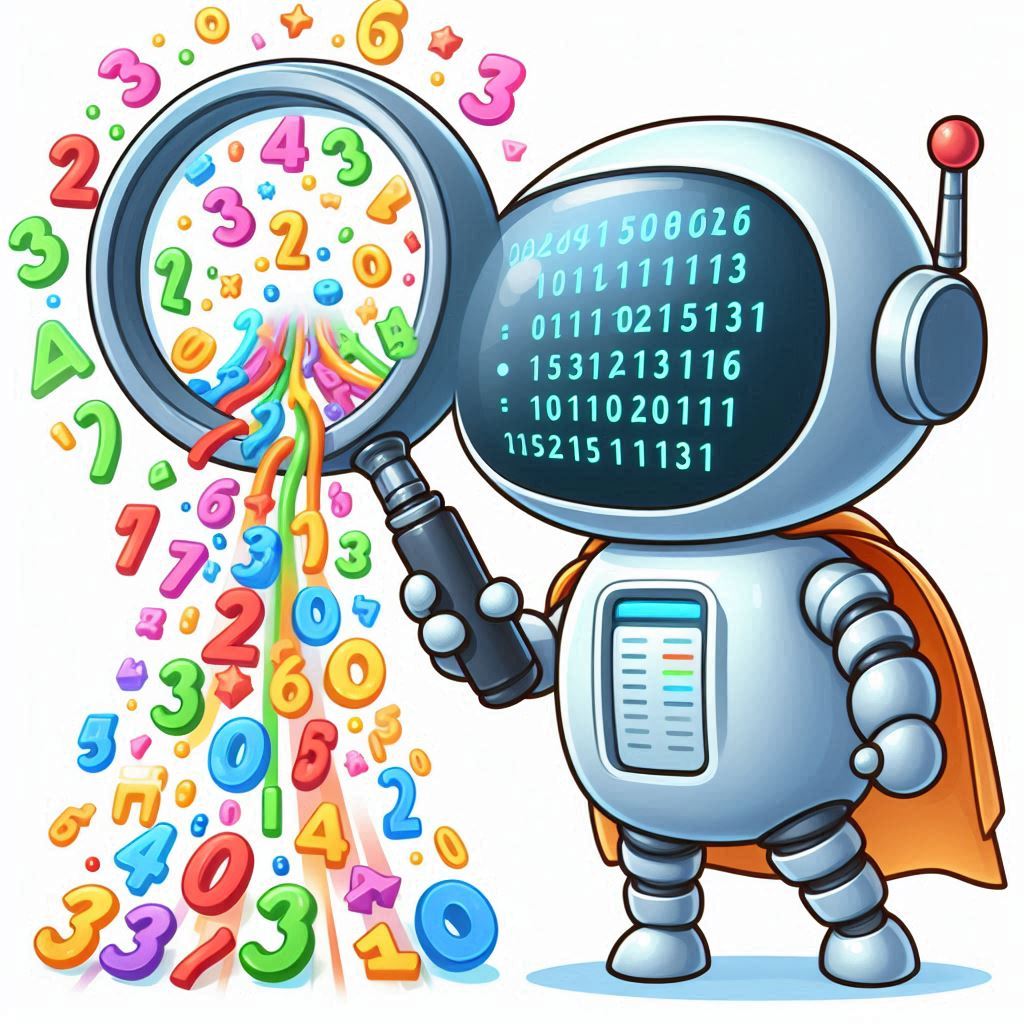
Stream Operators: >>
and <<
- Stream Extraction Operator (
>>
): When the binary operator>>
is used with an input stream object likecin
, it is called the stream extraction operator. It extracts data from the input stream and stores it in a variable. The left-side operand of>>
must be an input stream variable likecin
, and the right-side operand must be a variable that will hold the input data. - Stream Insertion Operator (
<<
): The binary operator<<
is called the stream insertion operator when used with an output stream object likecout
. It inserts data into the output stream, directing it to the output device. The left-side operand of<<
must be an output stream variable likecout
, and the right-side operand must be an expression or a manipulator that will be output.
Handling Input and Output with cin
and cout
- Skipping Whitespace: When inputting data into a variable using the
>>
operator,cin
automatically skips all leading whitespace characters (such as spaces, tabs, and newlines). - Including Header Files: To use
cin
andcout
in a program, it is essential to include the header file<iostream>
, which contains the necessary definitions.
Stream Functions and Their Uses
get
: Theget
function reads data on a character-by-character basis without skipping any whitespace characters. It is particularly useful when you need to process every character, including spaces.ignore
: Theignore
function skips a specified number of characters in the input stream or skips characters until a specified delimiter is found. It is useful for discarding unwanted data.putback
: Theputback
function puts the last character retrieved by theget
function back into the input stream. This is useful when you need to reconsider or reprocess a character.peek
: Thepeek
function returns the next character from the input stream without removing it, allowing you to inspect the character without consuming it.
Error Handling and Stream States
- Fail State: When invalid data is read into a variable (e.g., trying to read a string into an integer variable), the input stream enters the fail state, indicating that an error occurred during input. Once in the fail state, subsequent input operations will fail unless the error is cleared.
- Clearing the Fail State: Use the’ clear’ function to restore the input stream to a working state after an input failure. This function clears the error flags and allows further input operations.
Output Formatting with Manipulators
setprecision
: This manipulator formats the output of floating-point numbers to a specified number of decimal places, providing control over the precision of your numerical output.fixed
: Thefixed
manipulator forces floating-point numbers to be displayed in a fixed decimal format rather than scientific notation.showpoint
: Theshowpoint
manipulator ensures that floating-point numbers are always displayed with a decimal point, even if the number has no fractional part, and adds trailing zeros if necessary.setw
: Thesetw
manipulator formats the output of an expression within a specified number of columns. By default, this output is right-justified, but you can combine it with other manipulators likeleft
to achieve left-justified output.setfill
: Thesetfill
manipulator is used to fill any unused columns on an output device with a specified character other than a space, making it useful for formatting tables and other structured output.
Working with Files in C++
- File I/O with
fstream
: To perform input and output operations with files, you need to include the<fstream>
header file in your program. This header file contains the definitions forifstream
(input file stream) andofstream
(output file stream), which are used to read from and write to files, respectively. - Opening and Closing Files: When working with file streams, you must declare variables of type
ifstream
for input andofstream
for output. These streams must be opened using theopen
function and closed using theclose
function. For example:
ifstream inFile;
ofstream outFile;
inFile.open("input.txt");
outFile.open("output.txt");
// Perform file operations
inFile.close();
outFile.close();
- Stream Functions with File I/O: You can use stream functions like
>>
,<<
,get
,ignore
,peek
,putback
, andclear
with file stream variables just as you would with standard I/O streams.
Streams in C++ are potent tools that manage the data flow between programs and external sources or destinations. By mastering the use of streams, manipulators, and file I/O, create programs that efficiently handle input and output operations, whether interacting with users via the keyboard and screen or managing data in files. Understanding these concepts is crucial for writing robust and versatile C++ programs.
+ There are no comments
Add yours