TypeScript Background
TypeScript is an open-source programming language developed and maintained by Microsoft. It was first released in 2012 to address the shortcomings of JavaScript, especially for large-scale applications. TypeScript is a strict syntactical superset of JavaScript, meaning it builds on top of JavaScript by adding static typing, classes, interfaces, and other features that help developers write more structured and maintainable code. Any valid JavaScript code is also valid TypeScript code, making it easy for JavaScript developers to adopt TypeScript incrementally.
TypeScript is designed to help developers catch errors at compile-time rather than at runtime, which is a significant advantage for large codebases and teams. Its type system allows developers to define data types (e.g., string, number, boolean, arrays, custom types), enabling better tooling, autocompletion, and refactoring.
When to Use TypeScript
TypeScript is beneficial in various scenarios, particularly when working on large-scale applications or when you need more robust tooling and error checking.
- Large-Scale Applications: TypeScript is ideal for large projects where you want to ensure that types and interfaces are consistent throughout your codebase. Its static typing helps prevent errors as the project scales.
- Team Collaboration: In environments where multiple developers are working on the same project, TypeScript ensures that everyone follows the same type definitions, making it easier to collaborate and avoid errors.
- Tooling & IDE Support: TypeScript provides better tooling support in editors like Visual Studio Code, thanks to its static typing. Features like autocompletion, refactoring tools, and inline documentation make coding faster and reduce bugs.
- Legacy JavaScript Projects: TypeScript can be incrementally adopted in existing JavaScript projects. You can start by renaming
.js
files to.ts
and gradually adding type annotations where needed. - Libraries and Frameworks: Many popular JavaScript libraries and frameworks (e.g., Angular, React, Vue) have TypeScript support, offering developers an improved development experience when building frontend applications.
Compatibility with Other Languages and Frameworks
TypeScript is fully compatible with JavaScript and integrates well with a variety of libraries, frameworks, and back-end languages:
- JavaScript Frameworks (React, Angular, Vue.js): TypeScript is widely used in modern JavaScript frameworks. Angular, for example, is built with TypeScript, and both React and Vue have strong TypeScript support for type-safe component development.
- Node.js: TypeScript works seamlessly with Node.js for building both server-side and client-side applications. It can be compiled into JavaScript that runs in the Node.js environment.
- Back-End Languages (Python, Java, C#): Although TypeScript is primarily used for front-end development, it can interact with back-end services through REST APIs or GraphQL, regardless of the back-end language (Python, Java, C#, etc.).
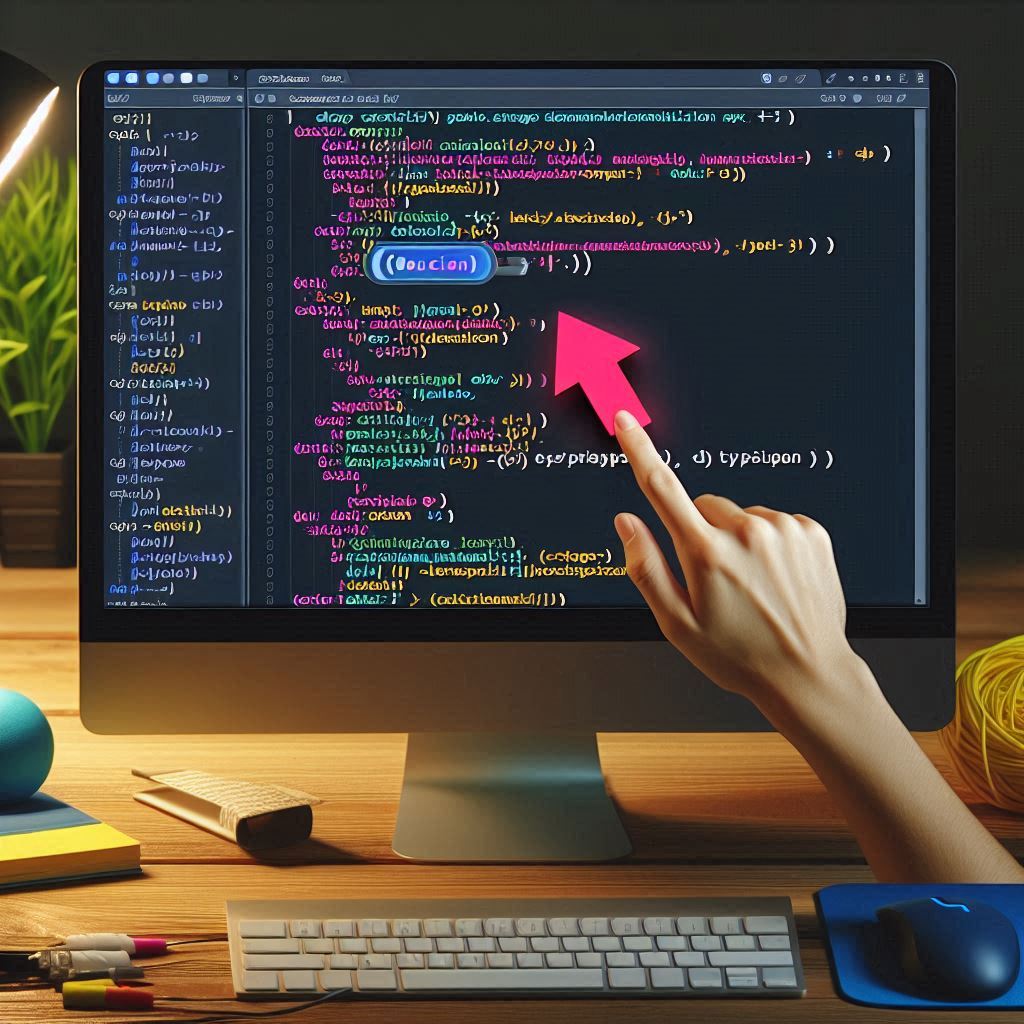
Examples of When TypeScript Is Used
- Static Typing and Interfaces:
TypeScript allows you to define types and interfaces to catch type-related errors during development.
interface User {
name: string;
age: number;
}
function greet(user: User) {
console.log(`Hello, ${user.name}!`);
}
const user = { name: 'John', age: 25 };
greet(user); // Works correctly
- Using TypeScript with React:
TypeScript can be integrated with React to provide type safety for props, state, and components.
import React from 'react';
interface ButtonProps {
label: string;
onClick: () => void;
}
const Button: React.FC<ButtonProps> = ({ label, onClick }) => {
return <button onClick={onClick}>{label}</button>;
};
export default Button;
- Error Checking at Compile Time:
TypeScript catches type errors at compile time, preventing bugs before the code is executed.
function add(a: number, b: number): number {
return a + b;
}
console.log(add(5, 10)); // Works fine
console.log(add(5, '10')); // Error: Argument of type 'string' is not assignable to parameter of type 'number'.
- Using TypeScript with Node.js:
TypeScript can be used to build back-end applications with Node.js, ensuring type safety.
import express from 'express';
const app = express();
app.get('/', (req, res) => {
res.send('Hello, TypeScript with Node.js!');
});
app.listen(3000, () => {
console.log('Server running on port 3000');
});
Benefits of TypeScript
- Type Safety: TypeScript helps catch type-related errors at compile time, which improves code quality and reduces the risk of runtime errors.
- Better Tooling: TypeScript provides advanced autocompletion, refactoring tools, and inline documentation in most code editors, thanks to its static typing.
- Improved Team Collaboration: In larger teams, TypeScript ensures that developers follow consistent type definitions, making it easier to maintain and scale projects.
- Ease of Refactoring: TypeScript makes refactoring easier by providing more accurate type information, helping developers avoid breaking changes.
- Modern JavaScript Features: TypeScript supports the latest ECMAScript (JavaScript) features and down-compiles them for older browsers, providing future-proof code.
When Not to Use TypeScript
- Small Projects: If you’re working on a small or simple JavaScript project, adding TypeScript might introduce unnecessary complexity.
- Learning Curve: For developers who are new to static typing, TypeScript’s type system can have a steep learning curve.
- Extra Compilation Step: TypeScript must be compiled into JavaScript, which adds an additional step to the build process.
Code Example: Defining Custom Types and Using Classes
// Defining a custom type
type Point = {
x: number;
y: number;
};
// Function to calculate distance between two points
function distance(pointA: Point, pointB: Point): number {
const dx = pointA.x - pointB.x;
const dy = pointA.y - pointB.y;
return Math.sqrt(dx * dx + dy * dy);
}
// Using classes in TypeScript
class Rectangle {
constructor(public width: number, public height: number) {}
area(): number {
return this.width * this.height;
}
}
const rect = new Rectangle(10, 5);
console.log(`Area of rectangle: ${rect.area()}`);
References
- TypeScript Documentation. (2024). TypeScript Handbook. Retrieved from https://www.typescriptlang.org/docs/
- Microsoft. (2024). TypeScript Overview. Retrieved from https://docs.microsoft.com/en-us/typescript/
- Mozilla Developer Network (MDN). (2024). TypeScript Guide. Retrieved from https://developer.mozilla.org/en-US/docs/Glossary/TypeScript
- React Documentation. (2024). Using TypeScript with React. Retrieved from https://reactjs.org/docs/static-type-checking.html#typescript
+ There are no comments
Add yours